Dependency inversion: write BETTER PYTHON CODE Part 2
Video Statistics and Information
Channel: ArjanCodes
Views: 21,734
Rating: 4.9921799 out of 5
Keywords: write better python code, dependency inversion principle, dependency injection, dependency inversion, solid design principles, visual studio, python tips, dependency inversion principle tutorial, dependency injection python, dependency inversion principle python, dependency inversion principle example, solid design principles python, python tips for beginners, python tips and tricks, python tips for competitive programming, Python tips and tricks advanced
Id: Kv5jhbSkqLE
Channel Id: undefined
Length: 9min 2sec (542 seconds)
Published: Thu Jan 28 2021
Please note that this website is currently a work in progress! Lots of interesting data and statistics to come.
Subscribed!! Thank you!
Subscribed. Clear and concise example. Thanks!
Subscribed, good job!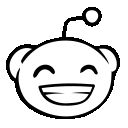
Thanks for the tutorial. A list of python modules designed to support this are here:
https://github.com/metaperl/python-oop/wiki/Dependency-Injection--&--Inversion-of-Control
Hi, you can use ctrl+F2 (or other combination of keys - it is also under context menu "change all occurrences") to refactor the names of variables, instead of changing them one at a time. I am referring to 6:13 in your video.
All in all great example how to do this, keep it up!
Love this! Anything that helps me be more efficient is a gem. So thank you!
A short description?